Table of Contents
ToggleProblem
Write a program for 8051 microcontroller to unpack a number stored in memory location 30h. Store the lower nibble in 31h and higher nibble in 32h.
Algorithm
Step 1: Load the number in accumulator.
Step 2: Mask the lower nibble to unpack.
Step 3: Store the unmasked lower digit in internal memory location.
Step 4: Load the number in accumulator again.
Step 5: Mask the higher nibble to unpack.
Step 6: Rotate 4 times or SWAP.
Step 7: Store the unmasked higher digit in internal memory location.
Step 8: Stop.
Flowchart
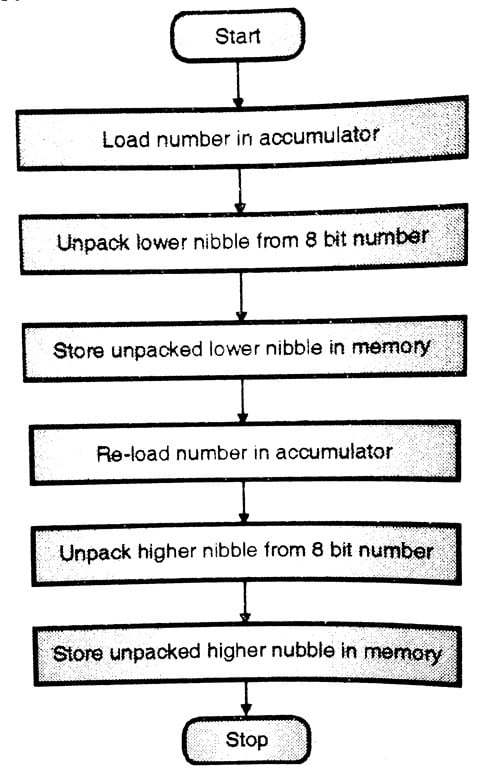
Program
MOV A, 30H ; Load the number from 30h in accumulator.
ANL A, #0FH ; AND content of accumulator with 0Fh to mask lower nibble.
MOV 31H, A ; Store the lower digit in 31h memory location.
MOV A, 30H ; Load the number in accumulator again.
ANL A, #0F0H ; AND content of accumulator with 0F0h to mask higher nibble.
SWAP A ; Exchange lower and higher nibble
MOV 32H, A ; Store the higher digit in 32h memory location.
LOOP: AJMP LOOP ; Stop.
Recent posts
Related posts:
- Assembler Directives in 8051 Microcontroller
- Features of 8051 Microcontroller
- Memory Organization of 8051 Microcontroller
- Addressing Modes in 8051 Microcontroller
- Instruction Set in 8051 Microcontroller
- Architecture of 8051 Microcontroller
- PSW Register in 8051 Microcontroller | Program Status Word
- Pin Configuration of 8051 Microcontroller
- The Stack and Stack Pointer in 8051 Microcontroller
- Stack Pointer Data Pointer and Program Counter in 8051 Microcontroller
- Functions of Timing and Control Unit of 8051
- Functions of Ports in 8051 Microcontroller
- Port Structure of 8051 Microcontroller
- Reset Circuit of 8051 Microcontroller
- Registers in 8051 Microcontroller
- Power Saving Mode in 8051 | 8051 Power Down and Idle Mode
- 8051 Microcontroller Family
- Program Development Steps in ALP
- Addition of Two 8-Bit Numbers in 8051 Microcontroller
- Addition of Two 8 Bit Numbers with Carry in 8051 Microcontroller
- 8051 Program for Addition of Two 16 Bit Numbers
- 8051 Program to Add an Array of Numbers
- 8051 Program for Subtraction of Two 16 bit Numbers
- 8051 Program to Multiply two 8 Bit numbers
- 8051 Program to Divide two 8 Bit numbers
- 8051 Program to Find the Largest Number in An Array
- 8051 Program to Find the Smallest Number in An Array Stored in Internal Memory
- Interrupts of 8051 Microcontroller
- 8051 Program to Arrange Numbers in Ascending Order
- 8051 Program to Find 2’s complement